Drupal 7 EntityFieldQuery shortcut class for nodes
By Emily Newsom
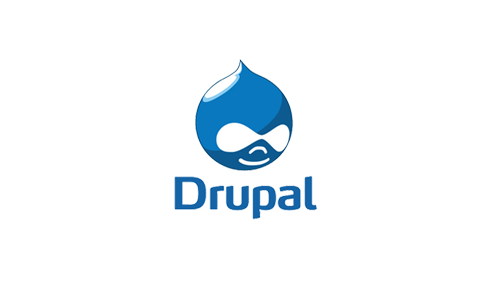
One of the big changes in Drupal 7 was to create another abstraction layer above nodes, users, etc. This abstraction layer allows one to access all of the various components as "entities". In Drupal 6, I always felt the push to make everything be a node, so this abstraction layer solves that problem--just treat everything as an entity and don't force the node issue.
So how do you query for entities? Like a lot of ORMs, Drupal now has its own query language--one can use EntityFieldQuery to query for any entity. Now if anyone has worked with EntityFieldQuery, it can be a bit (!) verbose. The problem I ran into is that 90% of the time I am looking for nodes only, so why do I need to deal with entities? Well, I threw together a subclass of EntityFieldQuery that only queries for nodes. Seems like a pretty obvious class to have in the core to speed up node queries, but I couldn't find anything of the sort so I wrote my own. Lets hope we get something like this in D8 before node_load_multiple's conditions get removed.
/* * NodeFieldQuery - An EntityFieldQuery object just for loading nodes because that's what we want 90% of the time. */ class NodeFieldQuery extends EntityFieldQuery { public function __contruct($type = null) { parent::__construct(); $this->entityCondition('entity_type', 'node'); if ($type) { $this->entityCondition('bundle', $type); } } public function load() { $result = $this->execute(); if (!isset($result['node'])) { return array(); } $nids = array_keys($result['node']); $nodes = entity_load('node', $nids); return $nodes; } } /* Usage */ $q = new NodeFieldQuery('project'); $q->fieldCondition('field_project_company', 'nid', $company->nid, '='); $projects = $q->load();
Not quite ready to take the plunge?
Subscribe if you're looking for help but aren't quite ready. We'll keep you informed of our availability and special promotions.